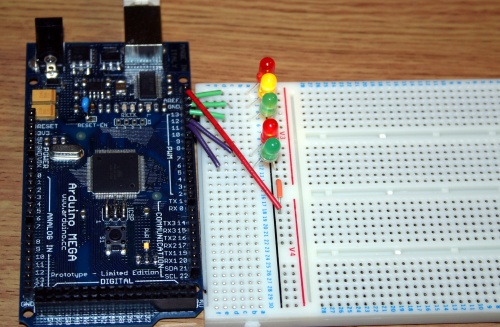
This is a bit enhanced version of the “hello world” blink application for Arduino: traffic lights emulator. The top three LEDs control car traffic and the bottom two are fore pedestrians.
int carRedPin = 13;
int carYellowPin = 12;
int carGreenPin = 11;
int pedestrianRedPin = 10;
int pedestrianGreenPin = 9;
// The setup() method runs once, when the sketch starts
void setup() {
pinMode(carRedPin, OUTPUT);
pinMode(carYellowPin, OUTPUT);
pinMode(carGreenPin, OUTPUT);
pinMode(pedestrianRedPin, OUTPUT);
pinMode(pedestrianGreenPin, OUTPUT);
}
// the loop() method runs over and over again,
// as long as the Arduino has power
void blink(int light)
{
digitalWrite(light, LOW);
delay(500);
digitalWrite(light, HIGH);
delay(500);
digitalWrite(light, LOW);
delay(500);
digitalWrite(light, HIGH);
delay(500);
digitalWrite(light, LOW);
delay(500);
digitalWrite(light, HIGH);
delay(500);
digitalWrite(light, LOW);
}
void loop()
{
digitalWrite(carRedPin, HIGH);
digitalWrite(pedestrianGreenPin, HIGH);
delay(3000);
blink(pedestrianGreenPin);
digitalWrite(pedestrianRedPin, HIGH);
digitalWrite(pedestrianGreenPin, LOW);
delay(1000);
digitalWrite(carYellowPin, HIGH);
delay(1000);
digitalWrite(carRedPin, LOW);
digitalWrite(carYellowPin, LOW);
digitalWrite(carGreenPin, HIGH);
delay(3000);
blink(carGreenPin);
digitalWrite(carYellowPin, HIGH);
delay(2000);
digitalWrite(carYellowPin, LOW);
digitalWrite(carRedPin, HIGH);
delay(1000);
digitalWrite(pedestrianRedPin, LOW);
}
The code above is straightforward: just enabling and disabling LED at certain time. Traffic lights in action:
[youtube]http://www.youtube.com/watch?v=f4jVitaSPGg[/youtube]